If youve ever written an interactive Java program, then you know that designing the GUI is not exactly a straightforward process. Basically, you have two choices. You can code each component of the interface in a Java source file piece by piece, or you can use one of the visual development tools. No matter which technique you use, theres a trade-off. Using the traditional method of coding every component means you need to have a thorough understanding of layout managers, containers, and action listeners, to name a few things. The traditional method is not easy. On the other hand, if you use an integrated development environment (IDE) such as VisualAge for Java, designing the GUI is very easy. But tying all the GUI components together into a working application is a lot more difficult. To successfully use one of these IDEs, you first need to have a thorough understanding of Java. If you ever have to go back and modify one of the Java source files generated by an IDE and you dont have the IDE available, then youll quickly discover that IDEs are nothing more than code generators, and the code they generate is unduly complex and nearly incomprehensible. Manual modifications are a nightmare. There needs to be a way to combine the flexibility of coding the pieces you want to code by hand with a simple GUI designer that lets you quickly create a Java GUI. If the IDE could make the generated code easy to understand and modify, two-thirds of the battle for programming in Java would be over.
Evidently, IBM also felt that something like this was needed. It created a new Java GUI design tool, GUI Builder, which lets you very rapidly build reusable Java GUI panels. However, before you can appreciate the ease GUI Builder will bring to your programming life, you must first understand why writing interactive Java programs the conventional way is so tedious. Since developing Java apps with an IDE is a monumental undertaking in and of itself, Ill skip any comparisons between that and the new GUI development tool. Ill leave it to you to try the IDEs for yourself.
Traditional GUI Design
Ill do something very simple. Ill create the Java GUI panel shown in Figure 1. This is a simple application that can be called from any DOS command line. As you can see, the only components on this panel are a label, a text box, and a button. Pretty basic, huh? At least it seems that way until you start digging into the code that makes it work. Ill show you what I mean. Figure 2 contains the code that displays the panel shown in Figure 1.
The Pieces
In Figure 2 (page 90), at Label A, Ive told Java to define a new Canvas object named GUICanvas. GUICanvas is a separate Java class and does nothing more than extend the Java Canvas class interface. It is the area where the GUI components will be displayed, inside a standard Windows frame. Label B tells Java that I want to use the Gridbag layout manager to define how components will be displayed in the GUI panel. Its up to you to decide which layout manager youll need to use. The C labels are used, in conjunction with the statement at Label B, to instantiate the Gridbag layout manager. Label D is used to instantiate the new canvas. The statements marked with Label E are used to define the GUI components, which, for this example, include a label, text field, and button. Each of these groups of instructions calls the addComponent method defined at Label H to actually add the components to the canvas. Because you have to specify the exact grid coordinates of each component in relation to all the other components, its easier to create one method to handle the placement and adding of the components to the canvas. Label F registers an actionListener to the button. This tells Java that some activity will be occurring with this GUI component and it should listen for this activity and take appropriate action. In this case, the action to be performed is to close the GUI panel. After all that, youre finally ready to display the GUI panel, which is what happens at Label G.
Piece of cake, right? Probably not, unless youve been programming in Java for quite some time and are used to it. And this is a really simple Java GUI! Imagine if you had a complex GUI to design and had to lay it out manually on your canvas. Yeesh! This is one of the reasons Java is still on the back burner of acceptance by traditional AS/400 green- screen programmers. After all, once youve used a tool like Screen Design Aid on the AS/400 to create display files, youre not going to be very accepting of something that makes you go back to graph paper and coordinate marking. Besides the drudgery of having to add all these components manually and defining the layout manager and canvas and on and on and on, all this stuff makes your code unnecessarily confusing. Instead of using this method, I will show you a tool that removes most of this complexity.
GUI Builder
GUI Builder is a Java-based WYSIWYG GUI design tool that was introduced with Modification 2 of the AS/400 Toolbox for Java. If you have Version 4, Release 4 of OS/400 or higher on your AS/400, then the Mod 2 version of the tool box resides there. I suggest, however, that you download the latest version of the AS/400 Toolbox for Java from the JTOpen site. (Go to www.as400.ibm.com/toolbox and follow the JTOpen links.)
GUI Builder is a visual design tool that lets you very rapidly and easily build Java GUI panels from scratch. It also lets you convert existing Windows Resource files (file type of RC) into an Extensible Markup Language (XML) representation that can be understood by GUI Builder. This lets you very quickly turn your existing Windows GUI definitions, those you created in C++ for example, into a format that can be understood by Java. In other words, in just a few minutes you can take a library of C++ GUI definitions you designed for Windows machines and make them completely platform-independent.
GUI Builder is able to accomplish all this by using a new technology developed by IBM called Panel Definition Markup Language (PDML). PDML is based on XML and is a way of describing GUI interfaces that are platform-independent. PDML, like XML or HTML, is a tag-based language. That is, each component of a GUI panel is defined with appropriate tags such as
Configuring and Running GUI Builder
The GUI Builder utility resides in a .jar file named UITOOLS.JAR. When you install the Mod 3 version of the AS/400 Toolbox for Java, this .jar file will reside in the same directory where all the AS/400 Toolbox for Java .jar files reside. To use GUI Builder, youre going to need to add the UITOOLS.JAR file to your PCs CLASSPATH. To do this, edit your AUTOEXEC.BAT file and add the following lines:
SET CLASSPATH=%CLASSPATH%;C:JT400LIBJT400.JAR
SET CLASSPATH=%CLASSPATH%;C:JT400LIBUITOOLS.JAR
SET CLASSPATH=%CLASSPATH%;C:JT400LIBJUI400.JAR
SET CLASSPATH=%CLASSPATH%;C:JT400LIBx4j400.jar.JAR
SET CLASSPATH=%CLASSPATH%;C:JT400LIBdata400.jar.JAR
In this example, Ive installed the Mod 3 version of the toolbox to a directory on my hard drive named JT400LIB. Youll need to change this path to match the location of the
.jar files on your own PC. The other piece youre going to need is a Java Development Kit such as the JDK
1.2 or JDK 1.3, available from Sun Microsystems at www.java.sun.com/j2se. Download and install this free JDK on your PC, following the instructions that come with it. Youll be making use of at least one command, Java, from that JDE when you use GUI Builder.
Once youve added the Mod 3 toolbox as well as the JDK from Sun Microsystems and modified your AUTOEXEC.BAT file, reboot your PC. When Windows restarts, your system should now be ready to work with GUI Builder. To start the utility, youll need to enter the following command at a DOS command line:
java com.ibm.as400.ui.tools.GUIBuilder -plaf Windows
This command starts GUI Builder and gives it the Windows look and feel. You also have the option of using the Metal or Motif look and feel.
When GUI Builder opens, click on the New Panel icon from the toolbar and youll see a panel somewhat similar to that shown in Figure 3. This figure duplicates the panel I created in Figure 1. Now you can add items such as buttons, text fields, labels, and list panels to the GUI panel by clicking on the appropriate component icon from the GUI Builder toolbar and dragging and dropping it anywhere on the panel. Contrast that method to the traditional method of positioning the various components by grid position, and the ease of a visual designer such as this becomes obvious.
Building Skeleton Code
Using GUI Builder, you also have the option of letting the tool create skeleton Java code for you for each component on your GUI panel. It works this way. Add a component such as a text field to the panel and then double-click on it to display its properties. Among the properties you can select are the components Data Class and Attribute. When you enter a name in the Data Class field, you are telling GUI Builder that this component will retrieve its data from a Java class of the name you give it. In other words, if you create a text field and enter a value of TextClass in the Data Class field of that text fields properties, GUI Builder will specify in the PDML file that the data for this component comes from a Java class named TextClass. The Attribute property lets you define the name of the methods GUI Builder will expect the class TextClass to contain. Each methodget, set, and so
onwill have the name getAttribute or setAttribute where Attribute will be replaced by the name you enter into the Attribute property field. So, assume that the Attribute property contains the name YourData. Your Java program would refer to the get value of this component with this form:
TextClass.getYourData();
Youll specify the Data Class and Attributes for each component that contains data on your panel. You can also indicate whether or not a given component will contain help information. If you enter something in the Generate Field Help property, and tell GUI Builder to do so, the tool will create HTML help using the names you supply in the properties panel. GUI Builder will also put the tags into the PDML document to point to the correct help file when the user selects HELP on that field. All you have to do is edit the HTML files to provide as much detail on each components HELP that you require. Pretty cool, huh?
When you are satisfied that you have designed your GUI panel just the way you want it, you are ready to save it. Before you do, click on the panel name and then click the Properties option from the View toolbar item in GUI Builder. In the properties panel that displays, youll find several options. Among them are the Generate Help, Generate Data Beans, and Generate Event Handlers options. If you set these properties to true when you save your work in GUI Builder, it will generate the HTML help file(s) and skeleton Java code for each component you set Data Class and Attribute properties for. After that, all you have to do is edit the Java source files created by GUI Builder to add the logic to retrieve and add the data to your GUI components and youre in business.
PDML File
The PDML source file generated by GUI Builder (Figure 4) is very easy to understand, unlike the Java code generated by a typical IDE such as VisualAge for Java. Although its not recommended, if you needed to modify the PDML file generated by GUI Builder manually, you could do it quite easily. Youd just have to ensure that the modifications you made conform to the PDML standards, which arent very difficult to understand. Another plus is that if you do make modifications to the PDML file, any Java class that references it can continue to use it without needing to be recompiled. That should save a lot of time.
OK, so now youve designed your new GUI, and youre ready to display it. How do you go about this? Actually, thats the easiest part of all. GUI Builder comes with some runtime Java classes called Panel Managers that parse the content of the PDML file into a GUI that displays on your screen.
Look at the code shown in Figure 5. There are only four lines of code your Java program needs to display a PDML GUI. These are the two import lines:
* pm = new PanelManager(...)
* pm.setVisible (true)
Thats it. Contrast that to all the code required by the traditional programming method. Of course, as with anything else, you can quickly get a lot more complex than this example.
For a complete tutorial on how to use the GUI Builder utility, refer to the online documentation for the AS/400 Toolbox for Java. The subsection titled GUI Builder contains a comprehensive set of help documentation that will get you up and running in a hurry with the GUI Builder utility.
Sending a Message
Developing applications in Java is something that all programmers need to learn how to do. The fact that most dont should be sending a signal to IBM, Sun Microsystems, and the other major players that perhaps the tools available for learning and developing Java apps are less than successful. IBM has made a very good step in addressing this problem by giving programmers the GUI Builder GUI design tool. Give it a try. With a little practice, youll find that creating interactive Java applications isnt as hard as you may have first thought.
REFERENCES AND RELATED MATERIALS:
IBM AS/400 Toolbox for Java and JTOpen:Downloads: www.as400.ibm.com/toolbox/downloads.htm
IBM AS/400 Toolbox for Java and JTOpen Web site: www.as400.ibm.com/toolbox
Sun Microsystems Java Development Kit 1.2 Web site: www.java.sun.com/j2se
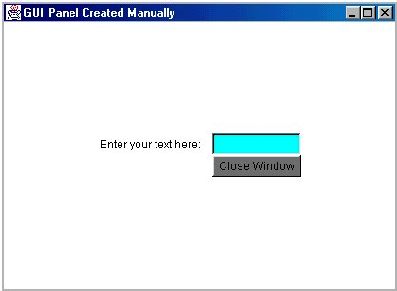
Figure 1: Creating a simple Java GUI can involve some intricate code.
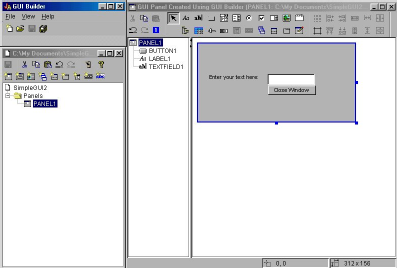
Figure 2: The traditional method of coding a Java GUI is not easy.
import java.awt.*;
import java.awt.event.*;
public class SimpleGUI extends Frame
implements ActionListener {
private GUICanvas c;
private GridBagLayout gbLayout;
private GridBagConstraints gbConstraints;
// Build the GUI Panel on the Canvas
public SimpleGUI (String s)
{ super (s);
gbLayout = new GridBagLayout();
setLayout( gbLayout );
// instantiate gridbag constraints
gbConstraints = new GridBagConstraints();
// set size of GUI Panel
c = new GUICanvas();
c.setSize( 400, 400 );
// Add Label
Label lb = new Label("Enter your text here:");
addComponent( lb, 1, 1, 2, 2 );
// Add Text Field
TextField tf = new TextField();
tf.setBackground (Color.cyan );
addComponent(tf, 2,4,10,2);
// Add button to close window
Button OK = new Button ("Close Window");
OK.setBackground( Color.gray );
addComponent(OK, 3,3,7,5);
// Register an action listener to the button to handle user actions
OK.addActionListener(this);
}
// Display Frame
public static void main(String[] args) {
SimpleGUI sGUI = new SimpleGUI("GUI Panel Created Manually");
sGUI.show();
}
// This method handles the action of the registered components
public void actionPerformed( ActionEvent e )
{
System.exit(0);
}
///////////////////////////////////////////////////////////////
// addComponent to canvas
///////////////////////////////////////////////////////////////
private void addComponent( Component c, int row, int column,
int width, int height )
{ // set gridx and gridy
gbConstraints.gridx = column;
gbConstraints.gridy = row;
// set gridwidth and gridheight
gbConstraints.gridwidth = width;
gbConstraints.gridheight = height;
// set constraints
gbLayout.setConstraints( c, gbConstraints );
add( c ); // add component to canvas
}
A C
C
D
E
F
G H
B E
E
}
Figure 3: GUI Builder lets you very rapidly develop Java GUIs.
Figure 4: The PDML file generated by GUI Builder is easy to understand for the example.
*
import com.ibm.as400.ui.framework.java.*;
import java.awt.Frame;
public class SimpleGUI2Src
{ public static void main(String[] args)
{
PanelManager pm = null;
try { pm = new PanelManager("SimpleGUI2", "PANEL1", null, new
Frame()); }
catch (DisplayManagerException e)
{
// Something didn't work, so display a message and exit
e.displayUserMessage(null);
System.exit(1);
}
// Display the panel
pm.setVisible(true);
// Exit the application
System.exit(0);
}
}
Figure 5: Contrast this Java code to that shown in Figure 1. Both display the same GUI.
LATEST COMMENTS
MC Press Online